Associated Types vs Generics in Traits
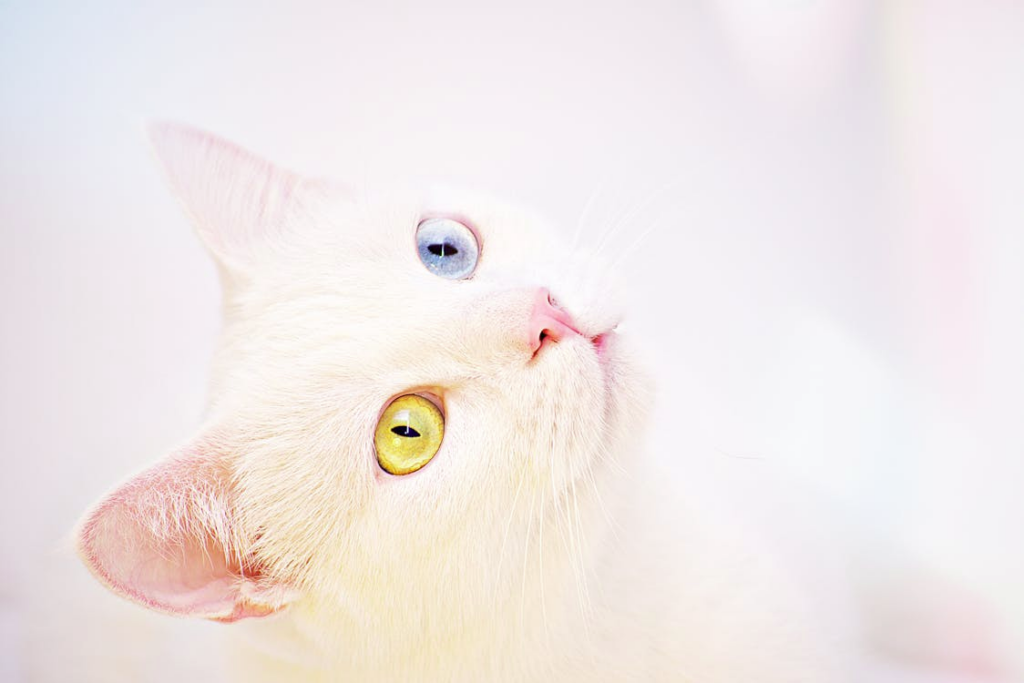
Instead of generic parameters in traits, Rust also allows associated types:
If you’re new to Rust the first time you see associated types may be with the Iterator trait
Example – the associated type is on line 2
trait Container {
type Item;
fn get(&self) -> &Self::Item;
}
struct Bucket<T> {
item: T,
}
impl<T> Container for Bucket<T> {
type Item = T;
fn get(&self) -> &Self::Item {
&self.item
}
}
fn main() {
let b = Bucket { item: 42 };
println!("{}", b.get());
}
Why use associated types?
- When a type is tied to a trait, it avoids specifying the generic type in every usage.
- It simplifies complex trait interactions.
The benefit may become clearer with more functions? Here you can see there is no need to specify <T>
on every trait usage.

Once you have Self::Item you can use it rather than T
trait Container {
type Item;
fn get(&self) -> &Self::Item;
fn update(&mut self, value: Self::Item);
}
struct Bucket<T> {
item: T,
}
impl<T> Container for Bucket<T> {
type Item = T;
fn get(&self) -> &Self::Item {
&self.item
}
fn update(&mut self, value: Self::Item) {
self.item = value;
}
}
impl<T> Bucket<T> {
fn new(value: T) -> Self {
Bucket { item: value }
}
}
fn main() {
let mut b = Bucket::new(42);
println!("Initial: {}", b.get());
b.update(100);
println!("Updated: {}", b.get());
}
Mastering Rust Generics: Associated Types vs Generic Traits
As we know, Rust’s powerful type system provides two approaches for working with generic data in traits: associated types and trait generics. Understanding when to use each can make your code more flexible and maintainable.
1. Understanding Generic Traits
A generic trait allows multiple implementations of a trait for different data types. This is useful when the type of data isn’t fixed for every implementation.
Example: File Storage with Generics
trait Storage<T> {
fn save(&self, item: T);
fn load(&self) -> T;
}
struct DiskStorage;
impl Storage<String> for DiskStorage {
fn save(&self, item: String) {
println!("Saving '{}' to disk.", item);
}
fn load(&self) -> String {
"Data from disk".to_string()
}
}
fn main() {
let disk = DiskStorage;
disk.save("My File".to_string());
println!("{}", disk.load());
}
Pros & Cons of Generics in Traits
โ
Works for multiple types (flexible).
โ Each implementation must specify the type, leading to more code.
2. Using Associated Types
An associated type defines a fixed type within a trait implementation. This is useful when the type logically belongs to the implementation.
Example: Cloud Storage with Associated Types
trait Storage {
type Data;
fn save(&self, item: Self::Data);
fn load(&self) -> Self::Data;
}
struct CloudStorage;
impl Storage for CloudStorage {
type Data = Vec<u8>; // Storing binary data
fn save(&self, item: Self::Data) {
println!("Uploading {} bytes to cloud.", item.len());
}
fn load(&self) -> Self::Data {
vec![1, 2, 3, 4] // Mocked binary data
}
}
fn main() {
let cloud = CloudStorage;
cloud.save(vec![10, 20, 30]);
println!("{:?}", cloud.load());
}
Pros & Cons of Associated Types
โ
More concise if the type is fixed per implementation.
โ Harder to switch types dynamically.
3. When to Use Each Approach?
Associated Types vs Generics in Traits
Feature | Generic Traits (trait Storage<T> ) | Associated Types (trait Storage { type Data; } ) |
---|---|---|
Flexibility | Can store multiple types | Fixed type per implementation |
Ease of Use | Must define type per use | More concise if type is known |
Complexity | More boilerplate | Simpler for single-use traits |
๐ Use generics if the trait should handle multiple types.
๐ Use associated types if the type is strongly linked to the implementation.
By mastering these concepts, you can write more powerful and expressive Rust code! ๐
“Associated types will force a single concrete type for each implementing struct while generics will allow any number of types for a given implementing struct”
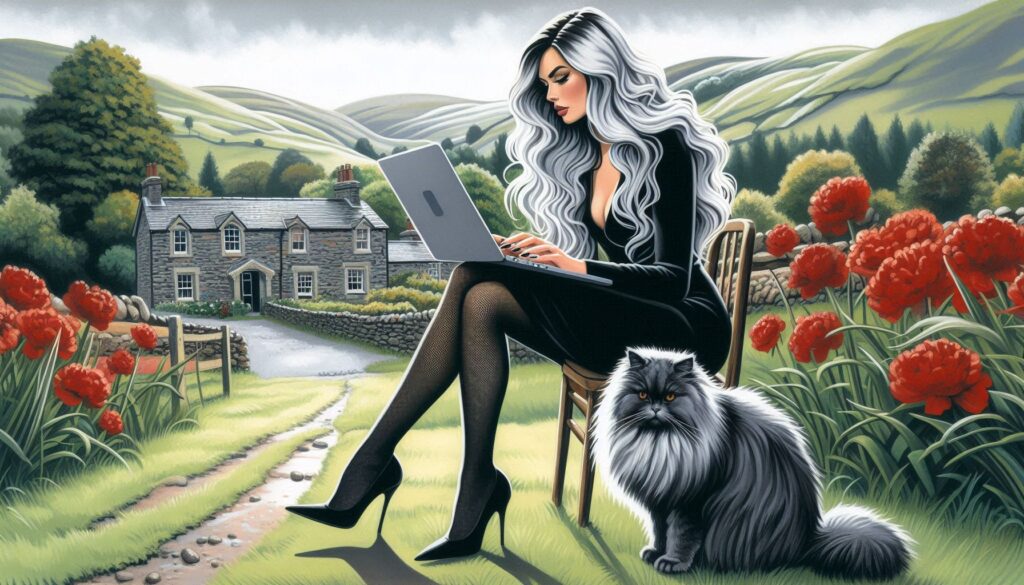